Guide to Batch Apex in Salesforce
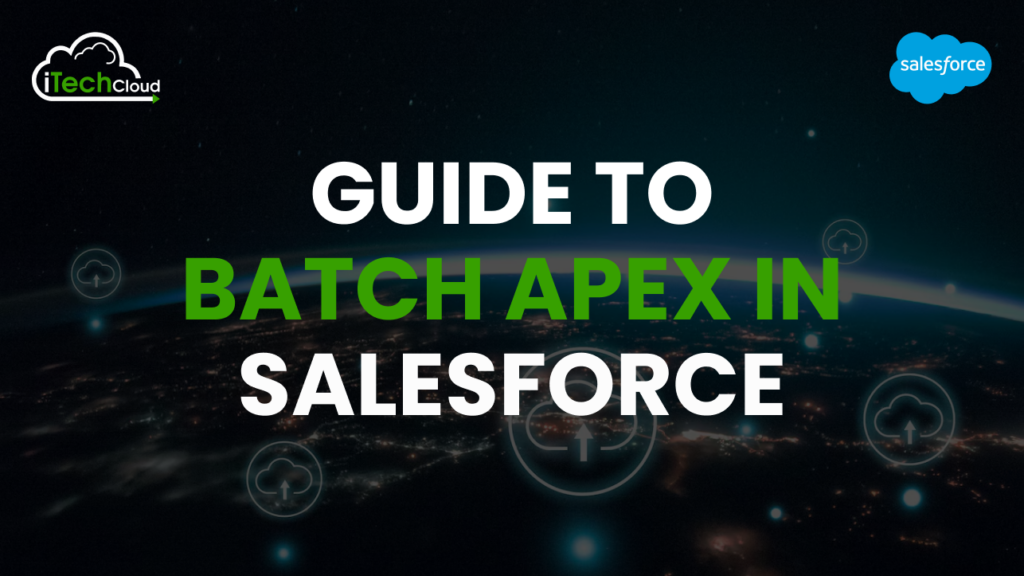
Salesforce is a powerful platform that enables businesses to streamline their processes and manage customer relationships effectively. One of its most notable features is the ability to process large amounts of data using Batch Apex in Salesforce. This guide will provide an in-depth overview of Batch Apex, covering its architecture, use cases, and best practices while optimizing search engines.
Table of Contents
What is Batch Apex in Salesforce?
Batch Apex is a Salesforce feature that allows developers to handle large-scale data operations asynchronously. Unlike standard triggers or synchronous Apex code, Batch Apex processes records in manageable chunks, ensuring efficient memory usage and avoiding governor limits.
Why Use Batch Apex in Salesforce?
Batch Apex in Salesforce is used to process large volumes of data efficiently by breaking the execution into manageable chunks. Salesforce enforces governor limits to ensure efficient use of shared resources, such as the number of database queries or DML operations in a single transaction. Batch Apex overcomes these limits by dividing the data into smaller batches, each processed as a separate transaction. This ensures that resource limits are adhered to while handling millions of records.
Batch Apex is particularly valuable when performing operations that involve processing or transforming extensive datasets. Examples include data cleansing, mass updates, system integrations, recalculations, or migrating data. Breaking tasks into smaller transactions provides robustness; if a batch fails, only that specific batch needs to be retried instead of reprocessing the entire dataset.
The Batch Apex framework includes three key methods start, execute, and finish. The start method gathers the dataset for processing, the execute method manages each batch, and the finish method performs post-processing tasks like sending notifications. Developers can also control the size of each batch (default is 200 records) to optimize processing performance.
Batch Apex can be scheduled to run asynchronously, allowing resource-intensive operations to occur in the background without affecting system performance for real-time users. It also supports stateful processing, enabling variables to persist across batches for complex operations.
Explanation of Methods in Batch Apex Salesforce
Batch Apex in Salesforce is a framework for processing large volumes of data in smaller, manageable transactions. It is built around three core methods: start
, execute
, and finish
, each playing a distinct role in the batch-processing lifecycle.
1. Start Method:
The start
method initializes the batch job by preparing the dataset to process. It runs once at the beginning of the job and returns either a SOQL query or an iterable object, defining the records to be processed. This method determines the input for the batch and sets the stage for chunking the data into smaller groups.
2. Execute Method:
The execute
method is the workhorse of Batch Apex. It processes each chunk of data (called a scope), which consists of up to 200 records by default, though this size can be customized. The logic for the desired operation, such as updates, calculations, or deletions, is implemented here. Each batch runs as a separate transaction, ensuring that errors in one batch do not impact others. This makes processing more resilient and efficient.
3. Finish Method:
After all batches are processed, the finish
method executes to handle final tasks like logging, sending notifications, or initiating additional jobs. This ensures that any necessary cleanup or follow-up actions are completed.
Key Features of Batch Apex in Salesforce
Batch Apex in Salesforce is a powerful feature that allows developers to process large volumes of data efficiently and asynchronously. It is particularly useful for handling complex operations on millions of records that exceed the limits of regular Apex. Below are the key features of Batch Apex in Salesforce that make it a crucial tool for developers.
1. Efficient Processing of Large Data Volumes
Batch Apex enables the processing of massive datasets by breaking them into smaller, manageable batches. This approach ensures that Salesforce’s governor limits, which restrict data processing, are not exceeded. By processing records in chunks, Batch Apex allows for better scalability and performance when dealing with large volumes of data.
2. Asynchronous Execution for Seamless Operations
Batch Apex is its asynchronous execution. This means that Batch Apex runs in the background, without interrupting the user experience. Developers can schedule or queue batch jobs to run at specific times, minimizing the impact on system performance and ensuring smooth user interactions.
3. Batchable Interface for Custom Implementation
Batch Apex jobs are implemented using the Database.Batchable
interface, which requires three key methods:
start
: Defines the data scope and prepares the records for processing by querying or iterating over them.execute
: Performs the data processing task on each batch of records.finish
: Executes post-processing tasks, such as sending notifications or logging results after all batches have been completed.
4. Customizable Batch Size for Flexibility
Salesforce allows developers to configure the batch size, with the default set at 200 records. The batch size can be adjusted based on the complexity of the task, system resources, and performance needs. Smaller batches reduce memory consumption, while larger batches speed up processing.
5. Robust Error Handling
Batch Apex includes robust error-handling mechanisms. If an error occurs in one batch, only that specific batch is rolled back, while other batches continue processing. This ensures minimal disruption and helps maintain data integrity.
6. Chaining and Stateful Batches
Batch Apex supports job chaining, where one batch job can trigger another upon completion. It also allows stateful processing, meaning the Database.Stateful
interface can be used to retain variable values across batch executions, enabling cumulative data processing.
7. Monitoring and Debugging Tools
Salesforce provides built-in tools to monitor batch job progress. Through the Apex Jobs page, developers can track job status, view logs, and identify potential issues. This makes debugging easier and helps ensure optimal performance.
Top Use Cases for Batch Apex in Salesforce
Batch Apex in Salesforce is a powerful tool designed for processing large volumes of data asynchronously. By breaking up large datasets into smaller, manageable chunks, Batch Apex enables developers to handle complex operations without exceeding system limits. Here are some common use cases where Batch Apex is particularly beneficial:
1. Data Cleanup and Transformation
Batch Apex is cleaning up and transforming large datasets. For instance, if your Salesforce database contains outdated, duplicate, or incorrect records, Batch Apex (Read More) can be used to identify and update those records in bulk. This is essential for maintaining data quality and consistency across the platform.
2. Data Import and Export
Batch Apex can efficiently process records in batches, ensuring that the operation is completed within the system’s governor limits. For example, Batch Apex can be used to import thousands of leads, contacts, or accounts, updating or inserting records into Salesforce in an optimized way. Similarly, Batch Apex can export data from Salesforce to external systems or databases.
3. Mass Email or Notifications
Batch Apex is an ideal solution for sending mass emails or notifications to large numbers of Salesforce users or contacts. For example, it can be used to send personalized email campaigns or system-generated alerts. Rather than sending all emails at once, Batch Apex allows you to process them in smaller batches, helping avoid hitting email limits and ensuring better performance.
4. Data Archiving and Deletion
Batch Apex is useful for managing historical data, such as archiving older records or deleting obsolete data. Salesforce’s data storage is limited, and running batch jobs to archive or delete unnecessary records can free up space for more relevant data. Batch Apex can identify records that need to be archived or deleted based on specific criteria, making it easier to automate this task on a large scale.
5. Integration with External Systems
Batch Apex is often used to integrate Salesforce with external systems. For example, it can synchronize large datasets between Salesforce and external databases, processing data in batches to ensure smooth and efficient data transfer. This is particularly useful when integrating Salesforce with ERPs, CRMs, or other enterprise systems that contain massive amounts of data.
6. Scheduled Data Maintenance
Batch Apex can be scheduled to run at specific times, making it ideal for routine data maintenance tasks, such as updating records, recalculating values, or processing new data entries. Scheduling Batch Apex jobs allows businesses to automate these tasks without impacting system performance during peak hours.
Best Practices for Batch Apex in Salesforce
Batch Apex in Salesforce is a key tool for processing large datasets asynchronously, helping developers overcome governor limits by dividing tasks into smaller, manageable chunks. To make your batch Apex processes efficient and scalable, it’s important to follow best practices. Here’s a summary of essential Batch Apex best practices:
1. Optimize SOQL Queries:
Always ensure that your SOQL queries are selective and return only the necessary data (Read More). Use indexed fields and filter conditions to avoid querying unnecessary records. This reduces the load on Salesforce servers and helps avoid hitting governor limits.
2. Choose the Right Batch Size:
Batch size plays a crucial role in performance. While Salesforce allows a batch size between 1 and 2000, a batch size of around 200 is typically recommended. This size strikes a balance between resource usage and efficiency. Adjust the batch size according to your processing needs and data complexity.
3. Implement Efficient Scope Handling:
Divide your data into smaller, manageable chunks by defining a proper scope. This ensures that each execution processes a small set of records at a time, thus improving performance and reducing the risk of exceeding governor limits.
4. Manage SOQL and DML Limits:
Each batch execution can only perform a limited number of SOQL queries and DML operations. To stay within these limits, try to optimize your code by minimizing the number of DML operations and queries. Combining operations in bulk and using aggregate queries can significantly improve efficiency.
5. Error Handling and Logging:
Proper error handling ensures smooth batch execution. Implement try-catch blocks to capture exceptions and log errors for troubleshooting. Logging progress allows you to track the batch’s performance and identify potential issues early.
6. Avoid Recursive Executions:
Recursive execution of batch jobs can lead to an unintended sequence of operations. To prevent this, use flags or control variables to avoid triggering additional batch processes during execution, which can potentially lead to errors or performance degradation.
7. Testing with Realistic Data:
It is essential to test your Batch Apex code with data that mirrors the production environment as closely as possible. This helps identify potential issues under realistic conditions and ensures that your code performs well with large datasets.
8. Consider Queueable Apex for Simpler Jobs:
For simpler tasks that don’t require the complexity of Batch Apex, consider using Queueable Apex. It provides an easier-to-implement, lightweight alternative for asynchronous processing.
Conclusion:
Batch Apex in Salesforce is a powerful tool for handling large volumes of data asynchronously. It allows developers to process records in manageable chunks, improving performance and reducing system timeouts. By dividing a large operation into smaller, more efficient batches, Batch Apex ensures that complex data operations are executed without overloading the system. It is ideal for processes like data cleansing, reporting, and large-scale integrations.